React native参考手册
组件:FlatList | Components: FlatList
FlatList
一个用于渲染简单,扁平列表的高性能界面,支持最方便的功能:
- Fully cross-platform.
- 可选水平模式。
- 可配置的可视性回调。
- 标题支持。
- 页脚支持。
- 分隔符支持。
- 拉到刷新。
- 滚动加载。
- ScrollToIndex支持。
如果您需要部分支持,请使用<SectionList>
。
最小例子:
<FlatList
data={[{key: 'a'}, {key: 'b'}]}
renderItem={({item}) => <Text>{item.key}</Text>}
/>
演示PureComponent
性能优化和避免错误的更复杂的示例。
- 通过绑定的
onPressItem
处理程序,道具将保持===
和PureComponent
防止浪费的重新呈现,除非实际id
,selected
或title
道具的变化,即使内部SomeOtherWidget
有没有这样的优化。
- 通过传递
extraData={this.state}
,FlatList
我们确保FlatList
自己将在state.selected
更改时重新呈现。没有设置这个道具,FlatList
不知道它需要重新渲染任何物品,因为它也是一个PureComponent
,并且道具比较不会显示任何变化。
-
keyExtractor
告诉列表使用id
s作为反应键。
class MyListItem extends React.PureComponent {
_onPress = () => {
this.props.onPressItem(this.props.id);
};
render() {
return (
<SomeOtherWidget
{...this.props}
onPress={this._onPress}
/>
)
}
}
class MyList extends React.PureComponent {
state = {selected: (new Map(): Map<string, boolean>)};
_keyExtractor = (item, index) => item.id;
_onPressItem = (id: string) => {
// updater functions are preferred for transactional updates
this.setState((state) => {
// copy the map rather than modifying state.
const selected = new Map(state.selected);
selected.set(id, !selected.get(id)); // toggle
return {selected};
});
};
_renderItem = ({item}) => (
<MyListItem
id={item.id}
onPressItem={this._onPressItem}
selected={!!this.state.selected.get(item.id)}
title={item.title}
/>
);
render() {
return (
<FlatList
data={this.props.data}
extraData={this.state}
keyExtractor={this._keyExtractor}
renderItem={this._renderItem}
/>
);
}
}
这是一个方便的包装<VirtualizedList>
,因此继承了ScrollView
其中未明确列出的道具(以及那些道具)以及以下注意事项:
- 当内容滚出渲染窗口时内部状态不会保留。确保所有数据都在项目数据或外部商店(如Flux,Redux或Relay)中捕获。
- 这
PureComponent
意味着如果props
保持浅浅平等,它不会重新渲染。确保你的renderItem
函数所依赖的所有东西都作为一个道具(egextraData
)传递,而不是===
在更新之后传递,否则你的UI可能不会在更改时更新。这包括data
prop和父组件状态。
- 为了约束内存并启用平滑滚动,内容将异步呈现在屏幕外。这意味着可以以比填充率更快的速度滚动,并立即看到空白内容。这是一种权衡,可以根据每个应用程序的需要进行调整,并且我们正在努力在幕后改进它。
- 默认情况下,列表
key
在每个项目上查找一个道具并将其用于React键。或者,您可以提供自定义keyExtractor
道具。
另外也包含ScrollView Props,除非它嵌套在另一个FlatList的方向相同。
道具
numColumns?:
方法
scrollToEnd(params?: object)
滚动到内容的末尾。没有getItemLayout
道具可能会很难过。
scrollToIndex(params: object)
滚动到指定索引处的项目,使其位于可见区域中,使得viewPosition
0位于顶部,1位于底部,0.5位于中间位置。viewOffset
是固定数量的像素来抵消最终的目标位置。
注意:不能在不指定getItemLayout
道具的情况下滚动到渲染窗口之外的位置。
scrollToItem(params: object)
需要线性扫描数据 - scrollToIndex
如果可能,请使用。
注意:不能在不指定getItemLayout
道具的情况下滚动到渲染窗口之外的位置。
scrollToOffset(params: object)
滚动到列表中的特定内容像素偏移量。
查看VirtualizedList的scrollToOffset
recordInteraction()
告诉列表发生了交互,这应该触发可视性计算,例如,如果waitForInteractions
为true并且用户没有滚动。这通常是通过点击项目或导航操作来调用的。
flashScrollIndicators()
瞬间显示滚动指示器。
组件:FlatList | Components: FlatList相关
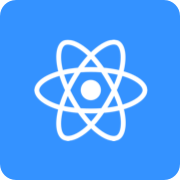
React Native 是一个 JavaScript 的框架,用来撰写实时的、可原生呈现 iOS 和 Android 的应用。
主页 | https://facebook.github.io/react-native/ |
源码 | https://github.com/facebook/react-native |
发布版本 | 0.49 |