React native参考手册
APIs
Linking
Projects with Native Code Only
本部分仅适用于react-native init
使用Create React Native App创建的项目或已经退出的项目。有关弹出的更多信息,请参阅创建React Native App存储库的指南。
Linking
为您提供了一个通用接口,可以与传入和传出应用程序链接进行交互
Basic Usage
Handling deep links
如果您的应用是从注册到您的应用的外部URL启动的,则您可以从任何需要的组件访问和处理该应用
componentDidMount() {
Linking.getInitialURL().then((url) => {
if (url) {
console.log('Initial url is: ' + url);
}
}).catch(err => console.error('An error occurred', err));
}
注意:有关如何在Android上添加对深度链接的支持的说明,请参阅启用应用程序内容的深层链接 - 为您的深层链接添加意向过滤器。
如果您希望在MainActivity的现有实例中接收意图,可以将launchMode
MainActivity 设置为singleTask
in AndroidManifest.xml
。查看<activity>
文档以获取更多信息。
<activity
android:name=".MainActivity"
android:launchMode="singleTask">
注意:在iOS上,您需要RCTLinking
按照此处所述的步骤链接到您的项目。如果您还想在应用执行过程中收听传入的应用链接,则需要将以下行添加到您的应用中*AppDelegate.m
:
// iOS 9.x or newer
#import <React/RCTLinkingManager.h>
- (BOOL)application:(UIApplication *)application
openURL:(NSURL *)url
options:(NSDictionary<UIApplicationOpenURLOptionsKey,id> *)options
{
return [RCTLinkingManager application:application openURL:url options:options];
}
如果您的目标是iOS 8.x或更高版本,则可以使用以下代码:
// iOS 8.x or older
#import <React/RCTLinkingManager.h>
- (BOOL)application:(UIApplication *)application openURL:(NSURL *)url
sourceApplication:(NSString *)sourceApplication annotation:(id)annotation
{
return [RCTLinkingManager application:application openURL:url
sourceApplication:sourceApplication annotation:annotation];
}
//如果您的应用程序使用通用链接,则还需要添加以下代码:
- (BOOL)application:(UIApplication *)application continueUserActivity:(NSUserActivity *)userActivity
restorationHandler:(void (^)(NSArray * _Nullable))restorationHandler
{
return [RCTLinkingManager application:application
continueUserActivity:userActivity
restorationHandler:restorationHandler];
}
然后在您的React组件上,您可以Linking
按照以下方式收听活动
componentDidMount() {
Linking.addEventListener('url', this._handleOpenURL);
},
componentWillUnmount() {
Linking.removeEventListener('url', this._handleOpenURL);
},
_handleOpenURL(event) {
console.log(event.url);
}
打开外部链接
To start the corresponding activity for a link (web URL, email, contact etc.), call
Linking.openURL(url).catch(err => console.error('An error occurred', err));
如果您想检查是否有任何已安装的应用程序可以事先处理给定的URL,您可以致电
Linking.canOpenURL(url).then(supported => {
if (!supported) {
console.log('Can\'t handle url: ' + url);
} else {
return Linking.openURL(url);
}
}).catch(err => console.error('An error occurred', err));
方法
constructor()
addEventListener(type, handler)
通过侦听url
事件类型并提供处理程序来添加处理程序来链接更改
removeEventListener(type, handler)
通过传递url
事件类型和处理程序来移除处理程序
openURL(url)
尝试url
用任何已安装的应用程序打开给定的。
您可以使用其他网址,例如位置(例如,Android上的“地理位置:37.484847,-122.148386”或iOS 上的“ http://maps.apple.com/?ll=37.484847,-122.148386 ”),联系人或任何可以使用已安装的应用程序打开的其他URL。
该方法返回一个Promise
对象。如果用户确认打开的对话框或URL自动打开,则承诺已解决。如果用户取消打开的对话框或没有为该URL注册的应用程序,该承诺将被拒绝。
注意:如果系统不知道如何打开指定的URL,则此方法将失败。如果您传递的是非http网址,则最好首先检查canOpenURL。
注意:对于网址,协议(“http://”,“https://”)必须相应设置!
canOpenURL(url)
确定安装的应用程序是否可以处理给定的URL。
注意:对于网址,协议(“http://”,“https://”)必须相应设置!
注意:从iOS 9开始,您的应用程序需要在LSApplicationQueriesSchemes
内部提供密钥,Info.plist
否则canOpenURL将始终返回false。
@param URL打开的URL
getInitialURL()
如果应用程序启动是由应用程序链接触发的,则会显示链接网址,否则会显示链接网址 null
注意:要支持Android上的深层链接,请参阅http://developer.android.com/training/app-indexing/deep-linking.html#handling-intents
APIs相关
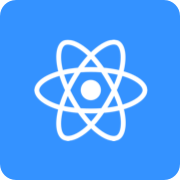
React Native 是一个 JavaScript 的框架,用来撰写实时的、可原生呈现 iOS 和 Android 的应用。
主页 | https://facebook.github.io/react-native/ |
源码 | https://github.com/facebook/react-native |
发布版本 | 0.49 |