React native参考手册
指南 | Guides
Navigating Between Screens
移动应用很少由一个屏幕组成。管理多个屏幕的呈现和转换通常由称为导航器的处理来处理。
本指南涵盖了React Native中可用的各种导航组件。如果您刚刚开始使用导航,则可能需要使用React Navigation。React Navigation提供了一个易于使用的导航解决方案,能够在iOS和Android上呈现常见堆栈导航和选项卡式导航模式。由于这是一个JavaScript实现,它在与诸如redux等状态管理库进行集成时提供了最大的可配置性和灵活性。
如果您只针对iOS,那么您可能还希望将NavigatorIOS作为一种以最少配置提供本机外观和感觉的方式,因为它为本机UINavigationController
类提供了一个包装。但是,该组件无法在Android上运行。
如果您希望在iOS和Android上实现本机外观和感觉,或者将React Native集成到已经本机管理导航的应用中,则以下库在两个平台上都提供本地导航:native-navigation,react-原生导航。
反应导航
社区导航解决方案是一个独立的库,它允许开发人员用几行代码设置应用程序的屏幕。
第一步是在你的项目中安装:
npm install --save react-navigation
然后,您可以快速创建一个包含主屏幕和个人资料屏幕的应用程序:
import {
StackNavigator,
} from 'react-navigation';
const App = StackNavigator({
Home: { screen: HomeScreen },
Profile: { screen: ProfileScreen },
});
每个屏幕组件都可以设置导航选项,例如标题标题。它可以使用navigation
道具上的动作创建者链接到其他屏幕:
class HomeScreen extends React.Component {
static navigationOptions = {
title: 'Welcome',
};
render() {
const { navigate } = this.props.navigation;
return (
<Button
title="Go to Jane's profile"
onPress={() =>
navigate('Profile', { name: 'Jane' })
}
/>
);
}
}
React导航路由器可轻松覆盖导航逻辑或将其集成到redux中。因为路由器可以互相嵌套,开发人员可以覆盖应用程序某个区域的导航逻辑,而无需进行大范围的更改。
React Navigation中的视图使用本机组件和Animated
库提供在本机线程上运行的60fps动画。此外,动画和手势可以轻松定制。
有关React Navigation的完整介绍,请按照React Navigation入门指南,或浏览其他文档,例如Navigator的Intro。
NavigatorIOS
NavigatorIOS
外观和感觉就像UINavigationController
,因为它实际上是建立在它的上面。
<NavigatorIOS
initialRoute={{
component: MyScene,
title: 'My Initial Scene',
passProps: { myProp: 'foo' },
}}
/>
像其他导航系统一样,NavigatorIOS
使用路线来表示屏幕,但有一些重要的区别。可以使用component
路径中的键来指定将要呈现的实际组件,并且可以在中指定应该传递给此组件的任何道具passProps
。“导航”对象作为道具组件自动传递,让你打电话push
和pop
需要。
由于NavigatorIOS
充分利用了原生的UIKit导航,它会自动渲染后退按钮和标题导航栏。
import React from 'react';
import PropTypes from 'prop-types';
import { Button, NavigatorIOS, Text, View } from 'react-native';
export default class NavigatorIOSApp extends React.Component {
render() {
return (
<NavigatorIOS
initialRoute={{
component: MyScene,
title: 'My Initial Scene',
passProps: {index: 1},
}}
style={{flex: 1}}
/>
)
}
}
class MyScene extends React.Component {
static propTypes = {
route: PropTypes.shape({
title: PropTypes.string.isRequired
}),
navigator: PropTypes.object.isRequired,
}
constructor(props, context) {
super(props, context);
this._onForward = this._onForward.bind(this);
}
_onForward() {
let nextIndex = ++this.props.index;
this.props.navigator.push({
component: MyScene,
title: 'Scene ' + nextIndex,
passProps: {index: nextIndex}
});
}
render() {
return (
<View>
<Text>Current Scene: { this.props.title }</Text>
<Button
onPress={this._onForward}
title="Tap me to load the next scene"
/>
</View>
)
}
}
查看NavigatorIOS
参考文档以了解有关此组件的更多信息。
指南 | Guides相关
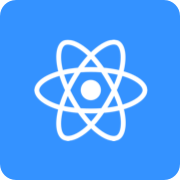
React Native 是一个 JavaScript 的框架,用来撰写实时的、可原生呈现 iOS 和 Android 的应用。
主页 | https://facebook.github.io/react-native/ |
源码 | https://github.com/facebook/react-native |
发布版本 | 0.49 |