JavaScript参考手册
函数 | Function
Method definitions
从ECMAScript 2015开始,在对象初始器中引入了一种更简短定义方法的语法,这是一种把方法名直接赋给函数的简写方式。
语法
var obj = {
property( parameters… ) {},
*generator( parameters… ) {},
async property( parameters… ) {},
async* generator( parameters… ) {},
// with computed keys:
[property]( parameters… ) {},
*[generator]( parameters… ) {},
async [property]( parameters… ) {},
// compare getter/setter syntax:
get property() {},
set property(value) {}
};
描述
该简写语法与ECMAScript 2015的getter和setter语法类似。
如下代码:
var obj = {
foo: function() {
/* code */
},
bar: function() {
/* code */
}
};
现可被简写为:
var obj = {
foo() {
/* code */
},
bar() {
/* code */
}
};
注意:简写语法使用命名函数而不是匿名函数(如…foo: function() {}
…)。命名函数可以从函数体调用(这对匿名函数是不可能的,因为没有标识符可以引用)。详细信息,请参阅function
。
生成器方法
生成器方法也可以用这种简写语法定义。使用它们时,
- 简写语法中的星号(*)必须出现在生成器名前,也就是说
* g(){}
可以正常工作,而g *(){}
不行。
- 非生成器方法定义可能不包含
yield
关键字。这意味着遗留的生成器函数也不会工作,并且将抛出SyntaxError
。始终使用yield
与星号(*)结合使用。
// Using a named property
var obj2 = {
g: function* () {
var index = 0;
while (true)
yield index++;
}
};
// The same object using shorthand syntax
var obj2 = {
* g() {
var index = 0;
while (true)
yield index++;
}
};
var it = obj2.g();
console.log(it.next().value); // 0
console.log(it.next().value); // 1
Async 方法
Async 方法也可以使用简写语法来定义。
// Using a named property
var obj3 = {
f: async function () {
await some_promise;
}
};
// The same object using shorthand syntax
var obj3 = {
async f() {
await some_promise;
}
};
Async 生成器方法
生成器方法也能成为 async.
var obj4 = {
f: async function* () {
yield 1;
yield 2;
yield 3;
}
};
// The same object using shorthand syntax
var obj4 = {
async* f() {
yield 1;
yield 2;
yield 3;
}
};
方法定义不是构造函数
所有方法定义不是构造函数,如果您尝试实例化它们,将抛出TypeError
。
var obj = {
method() {}
};
new obj.method; // TypeError: obj.method is not a constructor
var obj = {
* g() {}
};
new obj.g; // TypeError: obj.g is not a constructor (changed in ES2016)
示例
简单示例
var obj = {
a: 'foo',
b() { return this.a; }
};
console.log(obj.b()); // "foo"
计算属性名
速记语法还支持计算的属性名称。
var bar = {
foo0: function() { return 0; },
foo1() { return 1; },
['foo' + 2]() { return 2; }
};
console.log(bar.foo0()); // 0
console.log(bar.foo1()); // 1
console.log(bar.foo2()); // 2
规范
Specification |
Status |
Comment |
---|---|---|
ECMAScript 2015 (6th Edition, ECMA-262)The definition of 'Method definitions' in that specification. |
Standard |
Initial definition. |
ECMAScript 2016 (ECMA-262)The definition of 'Method definitions' in that specification. |
Standard |
Changed that generator methods should also not have a [Construct] trap and will throw when used with new. |
ECMAScript Latest Draft (ECMA-262)The definition of 'Method definitions' in that specification. |
Draft |
|
浏览器兼容性
Feature |
Chrome |
Edge |
Firefox (Gecko) |
Internet Explorer |
Opera |
Safari |
---|---|---|---|---|---|---|
Method definition shorthand |
39 |
(Yes) |
34 (34) |
No support |
26 |
No support |
Generator methods are not constructable (ES2016) |
? |
? |
43 (43) |
? |
? |
? |
Async methods |
? |
? |
52 (52) |
? |
? |
? |
Async generator methods |
? |
? |
55 (55) |
? |
? |
? |
Feature |
Android |
Chrome for Android |
Edge |
Firefox Mobile (Gecko) |
IE Mobile |
Opera Mobile |
Safari Mobile |
---|---|---|---|---|---|---|---|
Method definition shorthand |
No support |
No support |
(Yes) |
34.0 (34) |
No support |
No support |
No support |
Generator methods are not constructable (ES2016) |
? |
? |
? |
43.0 (43) |
? |
? |
? |
Async methods |
? |
? |
? |
52.0 (52) |
? |
? |
? |
Async generator methods |
? |
? |
? |
55.0 (55) |
? |
? |
? |
函数 | Function相关
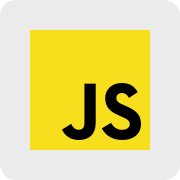
JavaScript 是一种高级编程语言,通过解释执行,是一门动态类型,面向对象(基于原型)的解释型语言。它已经由ECMA(欧洲电脑制造商协会)通过 ECMAScript 实现语言的标准化。它被世界上的绝大多数网站所使用,也被世界主流浏览器( Chrome、IE、FireFox、Safari、Opera )支持。JavaScript 是一门基于原型、函数先行的语言,是一门多范式的语言,它支持面向对象编程,命令式编程,以及函数式编程。它提供语法来操控文本、数组、日期以及正则表达式等,不支持 I/O,比如网络