JavaScript参考手册
类 | Classes
static
static 关键字为一个类定义了一个静态方法。
语法
static methodName() { ... }
描述
静态方法调用直接在类上进行,不能在类的实例上调用。静态方法通常用于创建实用程序函数。
调用静态方法
从另一个静态方法
在同一个类中的一个静态方法调用另一个静态方法,你可以使用this
关键字。
class StaticMethodCall {
static staticMethod() {
return 'Static method has been called';
}
static anotherStaticMethod() {
return this.staticMethod() + ' from another static method';
}
}
StaticMethodCall.staticMethod();
// 'Static method has been called'
StaticMethodCall.anotherStaticMethod();
// 'Static method has been called from another static method'
从类的构造函数和其他方法
静态方法不能直接在非静态方法中使用this
关键字来访问。你需要使用类名来调用它们:CLASSNAME.STATIC_METHOD_NAME()
或者将其作为构造函数的属性来调用该方法:this.constructor.STATIC_METHOD_NAME()
.
class StaticMethodCall {
constructor() {
console.log(StaticMethodCall.staticMethod());
// 'static method has been called.'
console.log(this.constructor.staticMethod());
// 'static method has been called.'
}
static staticMethod() {
return 'static method has been called.';
}
}
示例
下面的例子说明了这几点:
1.一个静态方法在一个类上是如何被实现的。
2.具有一个静态成员的一个类是可以被子类化 。
3.一个静态方法如何能被调用和不能被调用。
class Triple {
static triple(n) {
if (n === undefined) {
n = 1;
}
return n * 3;
}
}
class BiggerTriple extends Triple {
static triple(n) {
return super.triple(n) * super.triple(n);
}
}
console.log(Triple.triple()); // 3
console.log(Triple.triple(6)); // 18
var tp = new Triple();
console.log(BiggerTriple.triple(3));
// 81 (not affected by parent's instantiation)
console.log(tp.triple());
// 'tp.triple is not a function'.
规范
Specification |
Status |
Comment |
---|---|---|
ECMAScript 2015 (6th Edition, ECMA-262)The definition of 'Class definitions' in that specification. |
Standard |
Initial definition. |
ECMAScript Latest Draft (ECMA-262)The definition of 'Class definitions' in that specification. |
Living Standard |
|
浏览器兼容性
Feature |
Chrome |
Edge |
Firefox (Gecko) |
Internet Explorer |
Opera |
Safari |
---|---|---|---|---|---|---|
Basic support |
42.0 |
(Yes) |
45 (45) |
? |
? |
? |
Feature |
Android |
Edge |
Firefox Mobile (Gecko) |
IE Mobile |
Opera Mobile |
Safari Mobile |
Chrome for Android |
---|---|---|---|---|---|---|---|
Basic support |
No support |
(Yes) |
45.0 (45) |
? |
? |
? |
42.0 |
类 | Classes相关
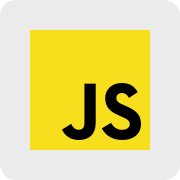
JavaScript 是一种高级编程语言,通过解释执行,是一门动态类型,面向对象(基于原型)的解释型语言。它已经由ECMA(欧洲电脑制造商协会)通过 ECMAScript 实现语言的标准化。它被世界上的绝大多数网站所使用,也被世界主流浏览器( Chrome、IE、FireFox、Safari、Opera )支持。JavaScript 是一门基于原型、函数先行的语言,是一门多范式的语言,它支持面向对象编程,命令式编程,以及函数式编程。它提供语法来操控文本、数组、日期以及正则表达式等,不支持 I/O,比如网络